Flag evaluation reasons
Read time: 25 minutes
Last edited: Oct 21, 2024
Overview
This topic explains how to use the flag evaluation reason feature to get more information about the flag variations LaunchDarkly serves to contexts or users.
You can find the evaluation reason for a specific context on its details page:
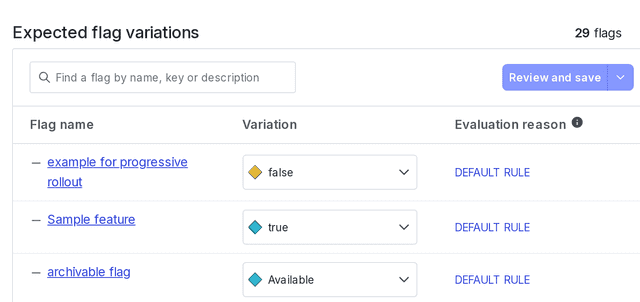
To learn more about how LaunchDarkly determines why a context or user receives a given flag variation, read Evaluation reasons in the SDK Concepts section. For additional guidance, read How to use the SDK's "evaluation reasons" feature to troubleshoot flag evaluation.
A context is a generalized way of referring to the people, services, machines, or other resources that encounter feature flags in your product. Contexts replace another data object in LaunchDarkly: "users." To learn more, read Contexts.
Creating contexts and evaluating flags based on them is supported in the latest major versions of most of our SDKs. For these SDKs, the code samples on this page include the two most recent versions.
Details about each SDK's configuration are available in the SDK-specific sections below.
Client-side SDKs
In client-side SDKs, you must enable an evaluation reason configuration option for this feature to work. The code samples below include this option. To learn more about configuration options, read Configuration.
This feature is available in the following client-side SDKs:
- .NET (client-side)
- Android
- C++ (client-side)
- Electron
- Flutter
- iOS
- JavaScript
- Node.js (client-side)
- React Native
- Roku
.NET (client-side)
Expand .NET (client-side) code sample
The VariationDetail
methods, such as BoolVariationDetail
, work the same as Variation
, but also provide additional "reason" information about how a flag value was calculated. For example, you can find out if the context was individually targeted for the flag or was matched by one of the flag's rules. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
var config = Configuration.Builder("mobile-key-123abc", ConfigurationBuilder.AutoEnvAttributes.Enabled).EvaluationReasons(true).Build();LdClient client = LdClient.Init(config, context);EvaluationDetail<bool> detail =client.BoolVariationDetail("bool-flag-key-123abc", false);// or StringVariationDetail for a string-valued flag, and so on.bool value = detail.Value;int? index = detail.VariationIndex;EvaluationReason reason = detail.Reason;
To learn more about the VariationDetail
methods, read EvaluationDetail
and BoolVariationDetail
. To learn more about the configuration option, read EvaluationReasons
.
Android
Expand Android code sample
The variationDetail
methods, such as boolVariationDetail
, work the same as variation
. They also provide additional "reason" information about how a flag value was calculated, such as if the context matched a specific rule. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
LDConfig ldConfig = new LDConfig.Builder(AutoEnvAttributes.Enabled).mobileKey("mobile-key-123abc").evaluationReasons(true).build();LDClient client = LDClient.init(this.getApplication(), ldConfig, context, secondsToBlock);EvaluationDetail<Boolean> detail =client.boolVariationDetail("flag-key-123abc", false);// or stringVariationDetail for a string-valued flag, etc.boolean value = detail.getValue();Integer index = detail.getVariationIndex();EvaluationReason reason = detail.getReason();
To learn more about the variationDetail
methods, read EvaluationDetail
and getVariationIndex
. To learn more about the configuration option, read evaluationReasons
.
Here is an example of how to access the details of a reason object:
void printReason(EvaluationReason reason) {switch (reason.getKind()) {case OFF:Timber.d("it's off");break;case FALLTHROUGH:Timber.d("fell through");break;case TARGET_MATCH:Timber.d("targeted");break;case RULE_MATCH:EvaluationReason.RuleMatch rm =(EvaluationReason.RuleMatch)reason;Timber.d("matched rule %d/%s",rm.getRuleIndex(),rm.getRuleId());break;case PREREQUISITE_FAILED:EvaluationReason.PrerequisiteFailed pf =(EvaluationReason.PrerequisiteFailed)reason;Timber.d("prereq failed: %s", pf.getPrerequisiteKey());break;case ERROR:EvaluationReason.Error e = (EvaluationReason.Error)reason;Timber.d("error: %s", e.getErrorKind());}// or, if all you want is a simple descriptive string:Timber.d(reason.toString());}
To learn more, read EvaluationReason
.
C++ (client-side)
Expand C++ (client-side) code sample
You can request and then programmatically inspect the reason for a particular feature flag evaluation.
The detail.Reason()
response is described in Evaluation reasons.
Here is an example:
auto detail = client.BoolVariationDetail("flag-key-123abc", false);if (detail.Value()) {std::cout << "Value was true!" << std::endl;} else {// it was false, let's find out why.if (auto reason = detail.Reason()) {// reason might not be present, so we have to checkstd::cout << "Value was false because of " << reason << std::endl;} else {std::cout << "No reason provided to explain why flag was false!" << std::endl;}}
To learn more, read EvaluationDetail
and BoolVariationDetail
.
Electron
Expand Electron code sample
The variationDetail
methods work the same as variation
. They also provide additional "reason" information about how a flag value was calculated. For example, you can find out if the context was individually targeted for the flag or was matched by one of the flag's rules. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
const { value, variationIndex, reason } = client.variationDetail('flag-key-123abc', false);
To learn more about the variationDetail
methods, read LDEvaluationDetail
and variationDetail
. To learn more about the configuration option, read LDEvaluationReason
.
Flutter
Expand Flutter code sample
The variationDetail
methods, such as boolVariationDetail
, work the same as variation
. They also provide additional "reason" information about how a flag value was calculated. For example, you can find out if the context was individually targeted for the flag or was matched by one of the flag's rules. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
To enable this functionality, set the evaluationReasons
configuration option to true
when you initialize the client.
Here is an example:
final config = LDConfig(CredentialSource.fromEnvironment,AutoEnvAttributes.enabled,dataSourceConfig: DataSourceConfig(evaluationReasons: true),);// initialize client and contextLDEvaluationDetail<bool> detail =client.boolVariationDetail('flag-key-123abc', false);// or stringVariationDetail for a string-valued flag, and so on.bool value = detail.value;int index = detail.variationIndex;LDEvaluationReason reason = detail.reason;
To learn more about the variationDetail
methods, read LDEvaluationDetail
and boolVariationDetail
. To learn more about the configuration option, read evaluationReasons
.
Here is an example of how to access the details of a reason object:
void printReason(LDEvaluationReason reason) {switch (reason.kind) {case LDKind.OFF:print("it's off");break;case LDKind.FALLTHROUGH:print('fell through');break;case LDKind.TARGET_MATCH:print('targeted');break;case LDKind.RULE_MATCH:print('matched rule: ${reason.ruleIndex} ${reason.ruleId}');break;case LDKind.PREREQUISITE_FAILED:print('prereq failed: ${reason.prerequisiteKey}');break;case LDKind.ERROR:print('error: ${reason.errorKind}');break;default: // LDKind.UNKNOWNprint('unknown service kind');}}
To learn more, read LDEvaluationDetail
.
iOS
Expand iOS code sample
The variationDetail
methods, such as boolVariationDetail
, work the same as the variation methods. They also provide additional "reason" information about how a flag value was calculated, such as if the user matched a specific rule. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
ldConfig.evaluationReasons = trueLDClient.start(config: ldConfig, context: context)let detail = client.boolVariationDetail(forKey: "flag-key-123abc", defaultValue: false);let value: Bool = detail.valuelet variationIndex: Int? = detail.variationIndexlet reason: [String: LDValue]? = detail.reason
To learn more about the variationDetail
methods, read LDEvaluationDetail
and boolVariationDetail
. To learn more about the configuration option, read LDConfig
.
JavaScript
Expand JavaScript code sample
The variationDetail
method lets you evaluate a feature flag with the same parameters you would for variation
. With variationDetail
, you receive more information about how the value was calculated.
The variation detail returns in an object containing both the result value and a "reason" object which tells you more information about the flag evaluation. For example, you can find out if the context was individually targeted for the flag or was matched by one of the flag's rules. It also indicates if the flag returned the default value due to an error. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
const options = { evaluationReasons: true };const client = LDClient.initialize('client-side-id-123abc', context, options);const detail = client.variationDetail('flag-key-123abc', false);const value = detail.value;const index = detail.variationIndex;const reason = detail.reason;
To learn more about the variationDetail
methods, read LDEvaluationDetail
and variationDetail
. To learn more about the configuration option, read evaluationReasons
.
Node.js (client-side)
Expand Node.js (client-side) code sample
The variationDetail
method lets you evaluate a feature flag with the same parameters you would for variation
. With variationDetail
, you receive more information about how the value was calculated.
The variation detail returns in an object that contains both the result value and a "reason" object which tells you more information about the flag evaluation. For example, you can find out if the user was individually targeted for the flag or was matched by one of the flag's rules. It also indicates if the flag returned the default value due to an error. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
const options = { evaluationReasons: true };const client = LDClient.initialize('client-side-id-123abc', user, options);const detail = client.variationDetail('flag-key-123abc', false);const value = detail.value;const index = detail.variationIndex;const reason = detail.reason;
To learn more about the variationDetail
method, read LDEvaluationDetail
and variationDetail
. To learn more about the configuration option, read evaluationReasons
.
React Native
Expand React Native code sample
The variationDetail
methods work the same way as the variation
methods, and also provide additional information about how a flag value was calculated. For example, you can find out if the context was individually targeted for the flag or was matched by one of the flag's rules. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export. To view this reason information, set the withReasons
configuration option to true
.
In React Native, there is a variation detail method for each type, such as boolVariationDetail
or stringVariationDetail
. In the React Native SDK version 10, there is also a hook for each type, such as useBoolVariationDetail
or useStringVariationDetail
.
Here is an example:
const { reason, value, variationIndex } = useBoolVariationDetail('flag-key-123abc', false);
To learn more about the variationDetail
methods, read LDEvaluationDetail
, useBoolVariationDetail
and boolVariationDetail
.
To learn more about the withReasons
configuration option, read LDOptions
.
The SDK also includes an untyped method to determine the variation of a feature flag and provide information about how the flag value was calculated. To learn more, read variationDetail
. We recommend using the strongly typed variation methods, such as boolVariationDetail
, which perform type checks and handle type errors.
Roku
Expand Roku code sample
For each variation type there is also an associated version that returns the reason a particular value was returned.
Here is an example:
config.setUseEvaluationReasons(true)details = launchDarkly.intVariationDetail("flag-key-123abc", 123)
These variation methods return an object containing the keys value
, reason
, and variationIndex
. The value
field is the result of the evaluation. The reason
field is an object that explains why the result happened, for example details about a rule match. The reason object will always contain a kind
field. Lastly the variationIndex
field contains the ID of the particular value returned. This field may be null.
Server-side SDKs
Unlike client-side SDKs, you do not need to enable an evaluation reason configuration option in server-side SDKs for this feature to work.
This feature is available in the following server-side SDKs:
- .NET (server-side)
- Apex
- C++ (server-side)
- Erlang
- Go
- Haskell
- Java
- Lua
- Node.js (server-side)
- PHP
- Python
- Ruby
- Rust
.NET (server-side)
Expand .NET (server-side) code sample
The VariationDetail
methods, such as BoolVariationDetail
, work the same as the Variation
methods, but also provide additional "reason" information about how a flag value was calculated. For example, you can find out if the context was individually targeted for the flag or was matched by one of the flag's rules. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
EvaluationDetail<bool> detail =client.BoolVariationDetail("flag-key-123abc", myContext, false);// or StringVariationDetail for a string-valued flag, etc.bool value = detail.Value;int? index = detail.VariationIndex;EvaluationReason reason = detail.Reason;
To learn more, read EvaluationDetail
, BoolVariationDetail
.
Here is an example of how to access the details of a reason object:
void PrintReason(EvaluationReason reason){switch (reason.Kind){case EvaluationReasonKind.OFF:Console.WriteLine("it's off");break;case EvaluationReasonKind.FALLTHROUGH:Console.WriteLine("fell through");break;case EvaluationReasonKind.TARGET_MATCH:Console.WriteLine("targeted");break;case EvaluationReasonKind.RULE_MATCH:var rm = reason as EvaluationReason.RuleMatch;Console.WriteLine("matched rule " + rm.RuleIndex + "/" + rm.RuleID);break;case EvaluationReasonKind.PREREQUISITE_FAILED:var pf = reason as EvaluationReason.PrerequisiteFailed;Console.WriteLine("prereq failed: " + pf.PrerequisiteKey);break;case EvaluationReasonKind.ERROR:var e = reason as EvaluationReason.Error;Console.WriteLine("error: " + e.ErrorKind);break;}// or, if all you want is a simple descriptive string:System.out.println(reason.ToString());}
To learn more, read EvaluationReason
.
Apex
Expand Apex code sample
By passing an LDClient.EvaluationDetail
object to a variation call you can programmatically inspect the reason for a particular evaluation.
Here is an example:
LDClient.EvaluationDetail details = new LDClient.EvaluationDetail();Boolean value = client.boolVariation(user, 'your.feature.key', false, details);/* inspect details here */if (details.getReason().getKind() == EvaluationReason.Kind.OFF) {/* ... */}
C++ (server-side)
Expand C++ (server-side) code sample
You can request and then programmatically inspect the reason for a particular feature flag evaluation.
The detail.Reason()
response is described in Evaluation reasons.
Here is an example:
auto detail = client.BoolVariationDetail(context, "flag-key-123abc", false);if (detail.Value()) {std::cout << "Value was true!" << std::endl;} else {// it was false, let's find out whyif (auto reason = detail.Reason()) {// reason might not be present, so we have to checkstd::cout << "Value was false because of " << reason << std::endl;} else {std::cout << "No reason provided to explain why flag was false!" << std::endl;}}
To learn more, read EvaluationDetail
.
Erlang
Expand Erlang code sample
The variation_detail
function is similar to the variation function, but also returns an explanation of the evaluation that you can inspect programmatically.
Here is an example:
Flag = ldclient:variation_detail(<<"flag-key-123abc">>, #{key => <<"context-key-123abc">>}, false)
Go
Expand Go code sample
The VariationDetail
methods, such as BoolVariationDetail
, work the same as Variation
, but also provide additional "reason" information about how a flag value was calculated. For example, you can find out if the context was individually targeted by the flag or was matched by one of the flag's rules. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
value, detail, err := client.BoolVariationDetail("flag-key-123abc", context, false)// or StringVariationDetail for a string-valued flag, etc.index := detail.VariationIndexreason := detail.Reason
To learn more, read EvaluationDetail
and BoolVariationDetail
.
Here is an example of how to access the details of a reason object:
import ("github.com/launchdarkly/go-sdk-common/v3/ldreason")func PrintReason(reason ldreason.EvaluationReason) {switch reason.GetKind() {case ldreason.EvalReasonOff:fmt.Println("it's off")case ldreason.EvalReasonFallthrough:fmt.Println("fell through")case ldreason.EvalReasonTargetMatch:fmt.Println("targeted")case ldreason.EvalReasonRuleMatch:fmt.Printf("matched rule %d/%s\n", r.GetRuleIndex(), r.GetRuleID())case ldreason.EvalReasonPrerequisiteFailed:fmt.Printf("prereq failed: %s\n", r.GetPrerequisiteKey())case ldreason.EvalReasonError:fmt.Printf("error: %s\n", r.GetErrorKind())}// or, if all you want is a simple descriptive string:fmt.Println(reason)}
To learn more, read EvaluationReason
.
If you are using OpenTelemetry, then instead of using the VariationDetail
method for each type, you must use the VariationDetailCtx
method for each type. For example, use BoolVariationDetailCtx
rather than BoolVariationDetail
. The methods are the same except that the VariationDetailCtx
methods also require a Go context parameter. This Go context is used in the hook implementation that provides OpenTelemetry support. To learn more, read OpenTelemetry.
Haskell
Expand Haskell code sample
The variationDetail
functions are similar to the variation
functions, but they also return an explanation of the evaluation that is programmatically inspectable.
Here is an example:
details :: IO (EvaluationDetail Bool)details = boolVariationDetail client "flag-key-123abc" context False
To learn more, read EvaluationDetail
and boolVariationDetail
.
Java
Expand Java code sample
The variationDetail
methods, such as boolVariationDetail
, work the same as variation
, but also provide additional "reason" information about how a flag value was calculated. For example, you can find out if the context was individually targeted for the flag or was matched by one of the flag's rules. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
import com.launchdarkly.sdk.*;EvaluationDetail<Boolean> detail =client.boolVariationDetail("flag-key-123abc", context, false);// or stringVariationDetail for a string-valued flag, and so on.boolean value = detail.getValue();int index = detail.getVariationIndex(); // will be < 0 if evaluation failedEvaluationReason reason = detail.getReason();
To learn more, read EvaluationDetail
and boolVariationDetail
.
Here is an example of how to access the details of a reason object:
void printReason(EvaluationReason reason) {switch (reason.getKind()) {case OFF:System.out.println("it's off");break;case FALLTHROUGH:System.out.println("fell through");break;case TARGET_MATCH:System.out.println("targeted");break;case RULE_MATCH:EvaluationReason.RuleMatch rm = (EvaluationReason.RuleMatch)reason;System.out.println("matched rule " + rm.getRuleIndex()+ "/" + rm.getRuleId());break;case PREREQUISITE_FAILED:EvaluationReason.PrerequisiteFailed pf =(EvaluationReason.PrerequisiteFailed)reason;System.out.println("prereq failed: " + pf.getPrerequisiteKey());break;case ERROR:EvaluationReason.Error e = (EvaluationReason.Error)reason;System.out.println("error: " + e.getErrorKind());}// or, if all you want is a simple descriptive string:System.out.println(reason.toString());}
To learn more, read EvaluationReason
.
Lua
Expand Lua code sample
By using the *VariationDetail
family of variation calls you can programmatically inspect the reason for a particular evaluation:
local details = client:boolVariationDetail(client, context, "flag-key-123abc", false);-- inspect details hereif details.reason == "FLAG_NOT_FOUND" thenend
To learn more, read boolVariationDetail
.
Node.js (server-side)
Expand Node.js (server-side) code sample
The variationDetail
method lets you evaluate a feature flag (using the same parameters as you would for variation
) and receive more information about how the value was calculated.
The variation detail is returned in an object that contains both the result value and a "reason" object which will tell you, for instance, if the context was individually targeted for the flag or was matched by one of the flag's rules. It will also indicate if the flag returned the default value due to an error. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
var detail = client.variationDetail('flag-key-123abc', context, false);var value = detail.value;var index = detail.variationIndex;var reason = detail.reason;
To learn more, read LDEvaluationDetail
and variationDetail
.
Here is an example of how to access the details of a reason object:
function printReason(reason) {switch(reason.kind) {case "OFF":console.log("it's off");break;case "FALLTHROUGH":console.log("fell through");break;case "TARGET_MATCH":console.log("targeted");break;case "RULE_MATCH":console.log("matched rule " + reason.ruleIndex + ", " + reason.ruleId);break;case "PREREQUISITE_FAILED":console.log("prereq failed: " + reason.prerequisiteKey);break;case "ERROR":console.log("error: " + reason.errorKind);break;}}
To learn more, read LDEvaluationReason
.
PHP
Expand PHP code sample
The variationDetail
method lets you evaluate a feature flag (using the same parameters as you would for variation
) and receive more information about how the value was calculated.
The variation detail is returned in an object that contains both the result value and a "reason" object which will tell you, for example, if the context was individually targeted for the flag or was matched by one of the flag's rules. It will also indicate if the flag returned the default value due to an error. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
$detail = $client->variationDetail("flag-key-123abc", $myContext, false);$value = $detail->getValue();$index = $detail->getVariationIndex();$reason = $detail->getReason();
To learn more, read EvaluationDetail
and variationDetail
.
Here is an example of how to access the details of a reason object:
function printReason($reason) {switch ($reason->getKind()) {case EvaluationReason::OFF:echo("it's off");break;case EvaluationReason::FALLTHROUGH:echo("fell through");break;case EvaluationReason::TARGET_MATCH:echo("targeted");break;case EvaluationReason::RULE_MATCH:echo("matched rule " . $reason->getRuleIndex() ."/" . $reason->getRuleId());break;case EvaluationReason::PREREQUISITE_FAILED:echo("prereq failed: " . $reason->getPrerequisiteKey());break;case EvaluationReason::ERROR:echo("error: " . $reason->getErrorKind());break;}// or, if all you want is a simple descriptive string:echo $reason;}
To learn more, read EvaluationReason
.
Python
Expand Python code sample
The variation_detail
method lets you evaluate a feature flag with the same parameters as you would for variation
. You can use this method to receive more information about how the value was calculated.
The variation detail is returned in an object that contains both the result value and a "reason" object which will tell you, for instance, if the context was individually targeted for the flag or was matched by one of the flag's rules. It will also indicate if the flag returned the default value due to an error. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
detail = client.variation_detail("flag-key-123abc", my_context, False)value = detail.valueindex = detail.variation_indexreason = detail.reason
To learn more, read EvaluationDetail
and variation_detail
.
Here is an example of how to access the details of a reason object:
def print_reason(reason):kind = reason["kind"]if kind == "OFF":print "it's off"elif kind == "FALLTHROUGH":print "fell through"elif kind == "TARGET_MATCH":print "targeted"elif kind == "RULE_MATCH":print "matched rule %d/%s" % (reason["ruleIndex"], reason["ruleId"])elif kind == "PREREQUISITE_FAILED":print "prereq failed: %s" % reason["prerequisiteKey"]elif kind == "ERROR":print "error: %s" % reason["errorKind"]
To learn more, read EvaluationDetail.reason
.
Ruby
Expand Ruby code sample
The variation_detail
method lets you evaluate a feature flag (using the same parameters as you would for variation
) and receive more information about how the value was calculated.
The variation detail is returned in an object that contains both the result value and a "reason" object which will tell you, for instance, if the context was individually targeted for the flag or was matched by one of the flag's rules. It will also indicate if the flag returned the default value due to an error. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
detail = client.variation_detail("flag-key-123abc", my_context, false)value = detail.valueindex = detail.variation_indexreason = detail.reason
To learn more, read EvaluationDetail
and variation_detail
.
Here is an example of how to access the details of a reason object:
def print_reason(reason)case reason[:kind]when "OFF"puts "it's off"when "FALLTHROUGH"puts "fell through"when "TARGET_MATCH"puts "targeted"when "RULE_MATCH"puts "matched rule #{reason[:ruleIndex]}/#{reason[:ruleId]}"when "PREREQUISITE_FAILED"puts "prereq failed: #{reason[:prerequisiteKey]}"when "ERROR"puts "error: #{reason[:errorKind]}"endend
To learn more, read EvaluationDetail.reason
.
Rust
Expand Rust code sample
The variation_detail
methods (for example, bool_variation_detail
) let you evaluate a feature flag, using the same parameters as you would for variation
, and receive more information about how the flag value was calculated. For example, you can find out if the context was individually targeted for the flag or was matched by one of the flag's rules. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
let detail = client.bool_variation_detail(&context, "flag-key-123abc", false);let value = detail.value;let index = detail.variation_index;let reason = detail.reason;
To learn more, read variation_detail
and bool_variation_detail
.
Here is an example of how to access the details of a reason object:
fn print_reason(reason: Reason) {match reason {Reason::Off => println!("it's off"),Reason::Fallthrough { .. } => println!("fell through"),Reason::TargetMatch => println!("targeted"),Reason::RuleMatch {rule_index,rule_id,..} => println!("matched rule {}/{}", rule_index, rule_id),Reason::PrerequisiteFailed { prerequisite_key } => {println!("prereq failed: {}", prerequisite_key)}Reason::Error { error } => println!("error: {:?}", error),};}
To learn more, read Reason
.
Edge SDKs
This feature is available for all of our edge SDKs:
Akamai
Expand Akamai code sample
The variationDetail
method lets you evaluate a feature flag using the same parameters as you would for variation
and receive more information about how the value was calculated.
The SDK returns the variation detail in an object that contains both the result value and a reason
object. These tell you more information. For example, they can tell you if the flag individually targeted the context, or if the context matched one of the flag's rules. It will also indicate if the flag returned the default value due to an error. You can examine the reason
data programmatically.
Here is an example:
const { value, variationIndex, reason } = await client.variationDetail(flagKey, context, false);
To learn more, read variationDetail
, LDEvaluationDetail
and LDEvaluationReason
.
The LDClient
also provides typed variation methods for type-safe usage in TypeScript: boolVariationDetail
, stringVariationDetail
, numberVariationDetail
, jsonVariationDetail
.
Cloudflare
Expand Cloudflare code sample
The variationDetail
method lets you evaluate a feature flag using the same parameters as you would for variation
and receive more information about how the value was calculated.
The SDK returns the variation detail in an object that contains both the result value and a "reason" object. These tell you, for instance, if the flag individually targeted the context or if the context matched one of the flag's rules. It will also indicate if the flag returned the default value due to an error. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
const { value, variationIndex, reason } = await client.variationDetail(flagKey, context, false);
To learn more, read variationDetail
, LDEvaluationDetail
and LDEvaluationReason
.
The LDClient
also provides typed variation methods for type-safe usage in TypeScript: boolVariationDetail
, stringVariationDetail
, numberVariationDetail
, jsonVariationDetail
.
Vercel
Expand Vercel code sample
The variationDetail
method lets you evaluate a feature flag using the same parameters as you would for variation
and receive more information about how the value was calculated.
The SDK returns the variation detail in an object that contains both the result value and a "reason" object. These tell you more information. For example, they can tell you if the flag individually targeted the context, or if the context matched one of the flag's rules. It will also indicate if the flag returned the default value due to an error. You can examine the "reason" data programmatically, or, if you capture detailed analytics events for flags, view it with Data Export.
Here is an example:
const { value, variationIndex, reason } = await client.variationDetail(flagKey, context, false);
To learn more, read variationDetail
, LDEvaluationDetail
and LDEvaluationReason
.
The LDClient
also provides typed variation methods for type-safe usage in TypeScript: boolVariationDetail
, stringVariationDetail
, numberVariationDetail
, jsonVariationDetail
.